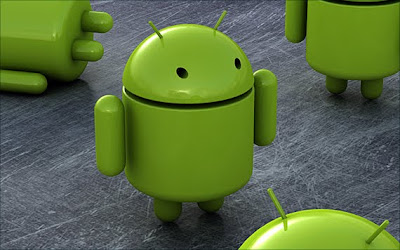
Hello again! Welcome to part 2 of this tutorial. Now, we will learn about Connecting UI control, Intent, Thread, and MediaPlayer
1. Connect UI to Code
If you want to take control with your Button, TextView, EditText, etc. You can use this instantiate:
Object UI name = (Object UI)findViewById(resource);
example:
Button myButton = (Button)findViewById(R.id.button1);
The example means that when you want to connect an UI component. You must create an Object to connect with your UI base on your component type. From the example: I want to connect button1 to code. So, I create a Button object named myButton that refers to the button1.
Note: You can explore the resource name in /gen/your package/R.java (Click on the triangle)
Look for the code from part1, I use Button to create Intent to navigate to another activity and run a new Thread to play sound at the same time.
btnCon = (Button)findViewById(R.id.btnCon);
btnCon.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// TODO Auto-generated method stub
}
});
I call the method setOnClickListener() from Button object and set listener to detect clicking for my btnCon button and then eclipse does auto implement onClick() method for me to take control the button.
2. Intent
Intent is the Navigator between two Activities. When Intent started, it stops current activity and calls new activity to display. When back button pressed it stops and destroys the new activity and restarts the previous activity to show again.
From part one when button was clicked:
Intent i = new Intent(getApplicationContext(),HelloAgain.class);
startActivity(i);
This will create an Intent name i and navigate to HelloAgain.class but it’s not fired yet then I use method startActivity(i) to fire the activity i to start HelloAgain Activity
3.Thread
At the same time with 2. I create a new Thread to play a sound. Thread is the thing that run method or make method works. In Java the method main() was run by main thread, but we can create a new thread to parallel run another method at the same time with main() method. This is useful with a method that consume time to wait. Imagine to when you make your breakfast if you cooking like a single thread program you may follow this step -- make toasts -- wait -- boil eggs -- wait -- boil water -- wait -- make coffee, so on. But if you do it in Multi-Thread you can make toasts, boil water and egg in the same time and only wait for make a coffee.
new Thread(new Runnable() {
public void run() {
}
}).start();
To start a new thread I create a runnable thread and call start() method to start a new thread. you can add method in run()
4. Media Player
Like it’s name, Media Player is for play medias. It can play both video and audio but at this time I will only show you only audio playing.
MediaPlayer mp = MediaPlayer.create(getApplicationContext(), R.raw.ring);
Simple to use, I create an Object in MediaPlayer type and name it as mp. Next, I connect it to resource file in /res/raw/ring.mp3 (eclipse will auto generate code to R.raw.ring) and then, my mp is ready to use
mp.start();
To use the mp, just call start() method and the sound will be play, nice and easy!!
note: the code mp.releash(); is use for free resource if mp is already have data. This method uses to free memory for system performance.
This tutorial is end. If you have any question please fell free to ask me. And... yeah.. sorry to my bad English skill...
No comments:
Post a Comment